Gameplay mechanics are the foundational rules, systems, and interactions that define how a video game operates. They dictate how players engage with the game world, interact with objects and characters, and progress through the game.
Core Mechanics
These are the fundamental actions and interactions that players engage in throughout the game. They often define the game’s genre and primary objectives. For example, in a first-person shooter, core mechanics include aiming, shooting, and reloading.
Physics and Movement
Gameplay mechanics govern how characters and objects move and interact with the game world. This includes aspects like gravity, friction, acceleration, and collision detection. Realistic physics can enhance immersion, while exaggerated physics can create unique gameplay experiences.
Object Interactions
Mechanics related to how players interact with objects within the game world. This can include picking up, using, combining, or manipulating items. Object interactions are crucial for puzzle-solving and progression.
Combat and Combat Mechanics
In action-oriented games, combat mechanics are central. These encompass combat actions, weapon types, damage calculations, health and damage systems, and enemy AI behavior. Combat mechanics can vary widely, from turn-based combat to real-time action.
Puzzle-Solving
Puzzle mechanics present challenges that require players to use logic, problem-solving skills, and in-game knowledge to progress. Puzzle mechanics may involve pattern recognition, spatial reasoning, or decoding clues.
Character Abilities and Progression
Many games feature character progression mechanics, where players can improve their character’s skills, attributes, or equipment over time. This adds depth and a sense of achievement to the gameplay.
Environmental Mechanics
These involve how the game environment affects gameplay. Environmental mechanics can include dynamic weather, day-night cycles, destructible terrain, or changing level layouts.
Ai Behaviour
For games with AI-controlled characters or enemies, AI behavior mechanics dictate how these entities react to the player’s actions. AI can range from simple scripted behaviors to complex learning algorithms.
Resource Management
Some games involve resource management mechanics, where players must allocate resources (e.g., currency, items, time) strategically to achieve their goals. This is common in strategy and simulation games.
Choice and Consequence
Games with branching narratives often feature choice and consequence mechanics. Player decisions can impact the story, character relationships, and game outcomes.
Time and Progression
Some games incorporate time-based mechanics, where events occur at specific times or within a time limit. Time mechanics can add urgency and challenge to gameplay.
Feedback and User Interface
Effective user interface (UI) and feedback mechanics provide players with information about their progress, objectives, and game state. Clear feedback enhances the player experience.
Economy and Trading
Games with in-game economies have mechanics related to buying, selling, and trading items. These mechanics are prevalent in simulation and role-playing games.
Stealth and Detection
Stealth mechanics govern how players can avoid or engage with enemy detection. This includes mechanics like line of sight, sound propagation, and stealth takedowns.
Minigames and Side Activities
Some games feature minigames or side activities that offer a diversion from the main gameplay loop. These can include card games, racing challenges, or crafting mechanics.
GAMEPLAY FRAMEWORK
Playlist
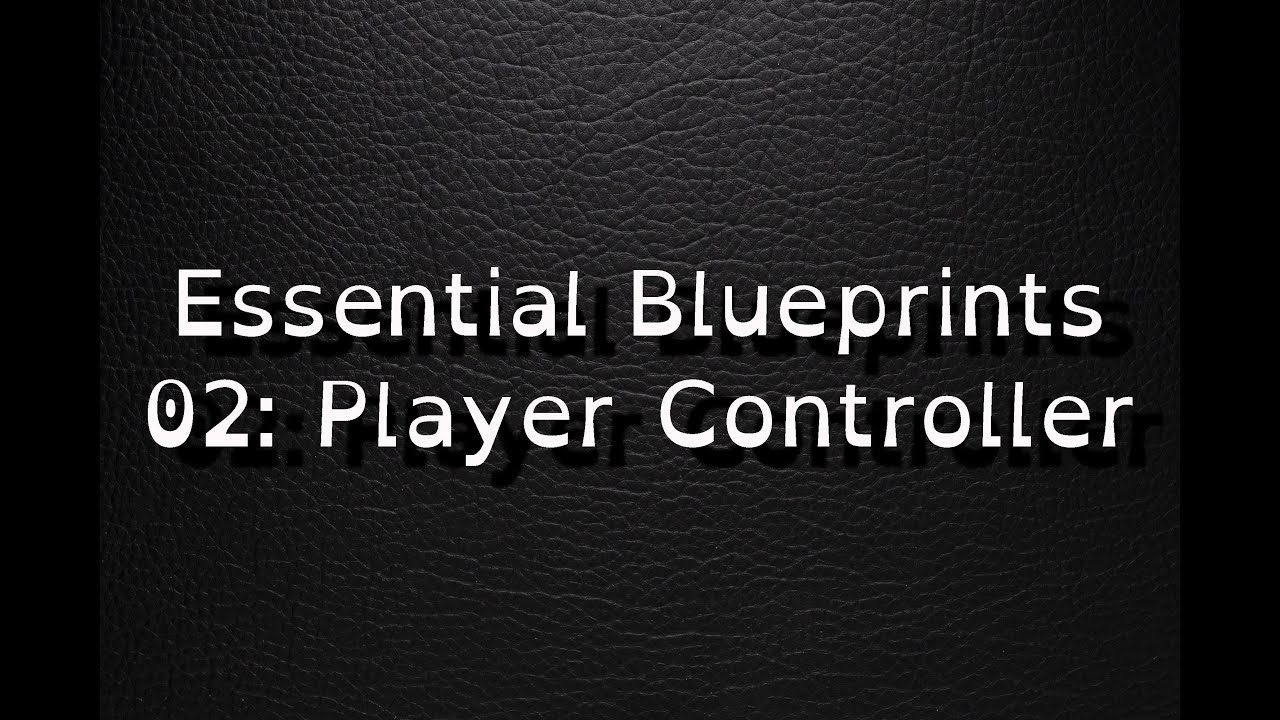
14:05
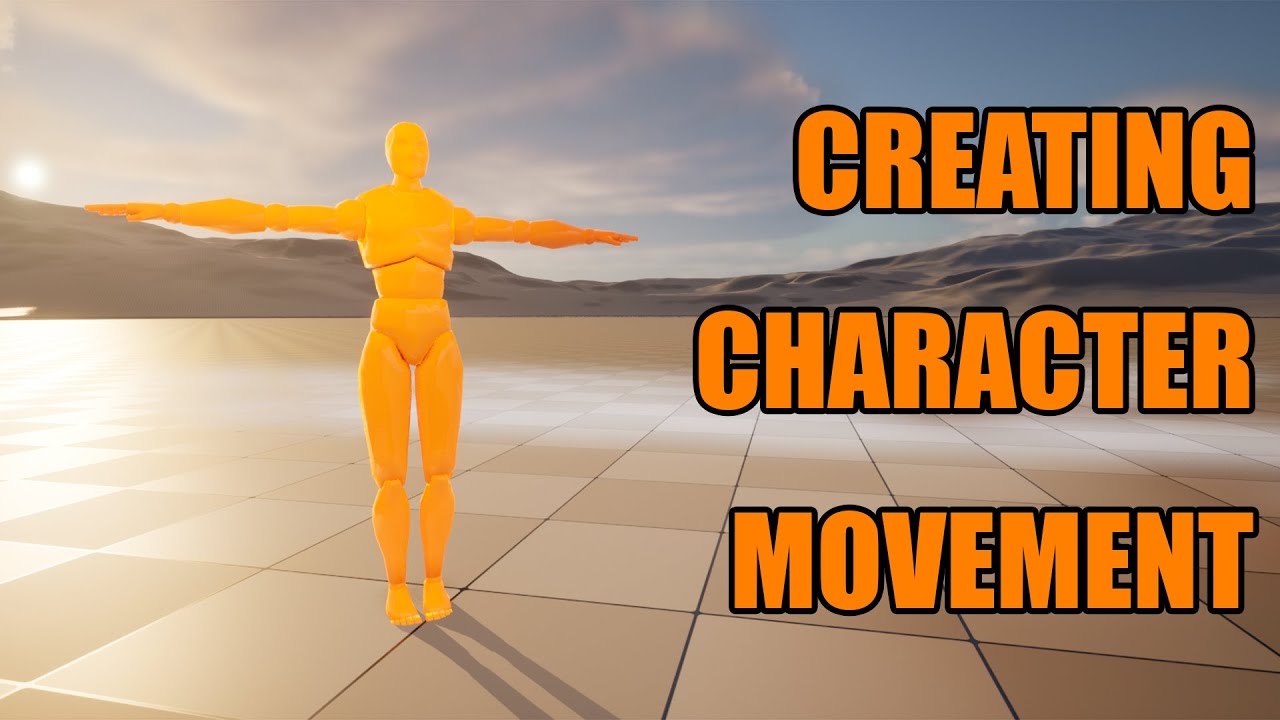
16:43
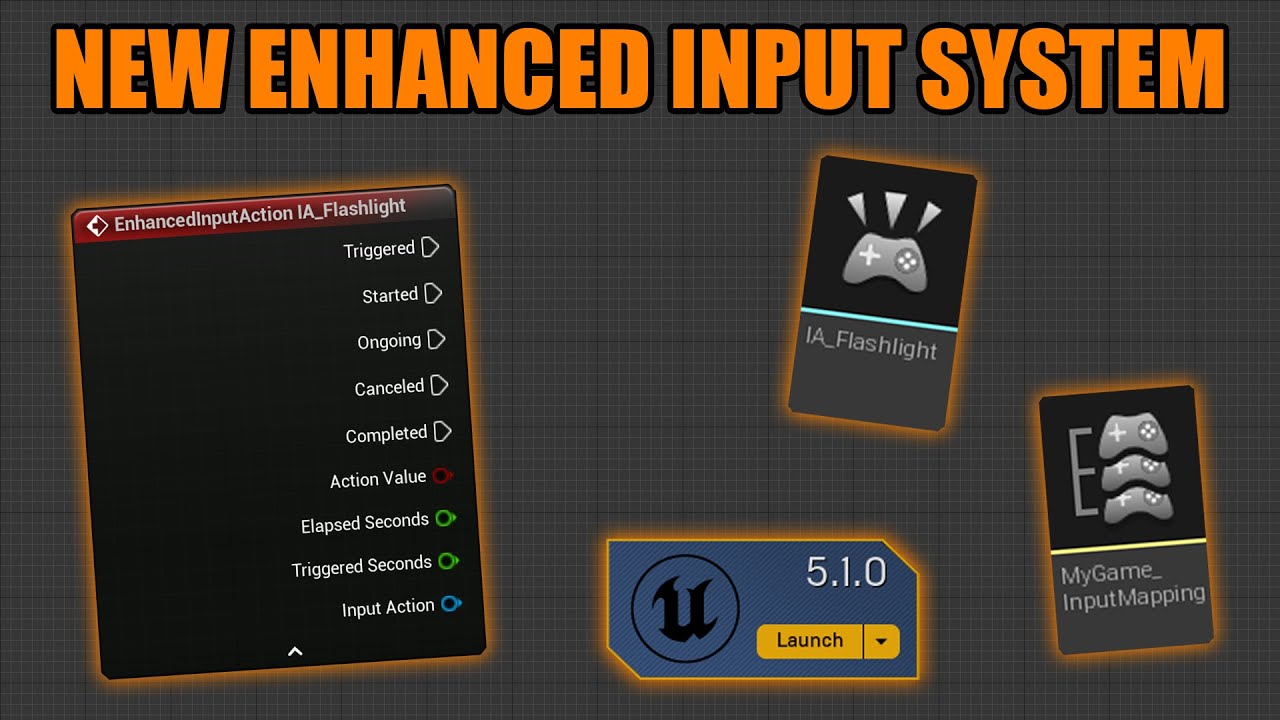
11:05
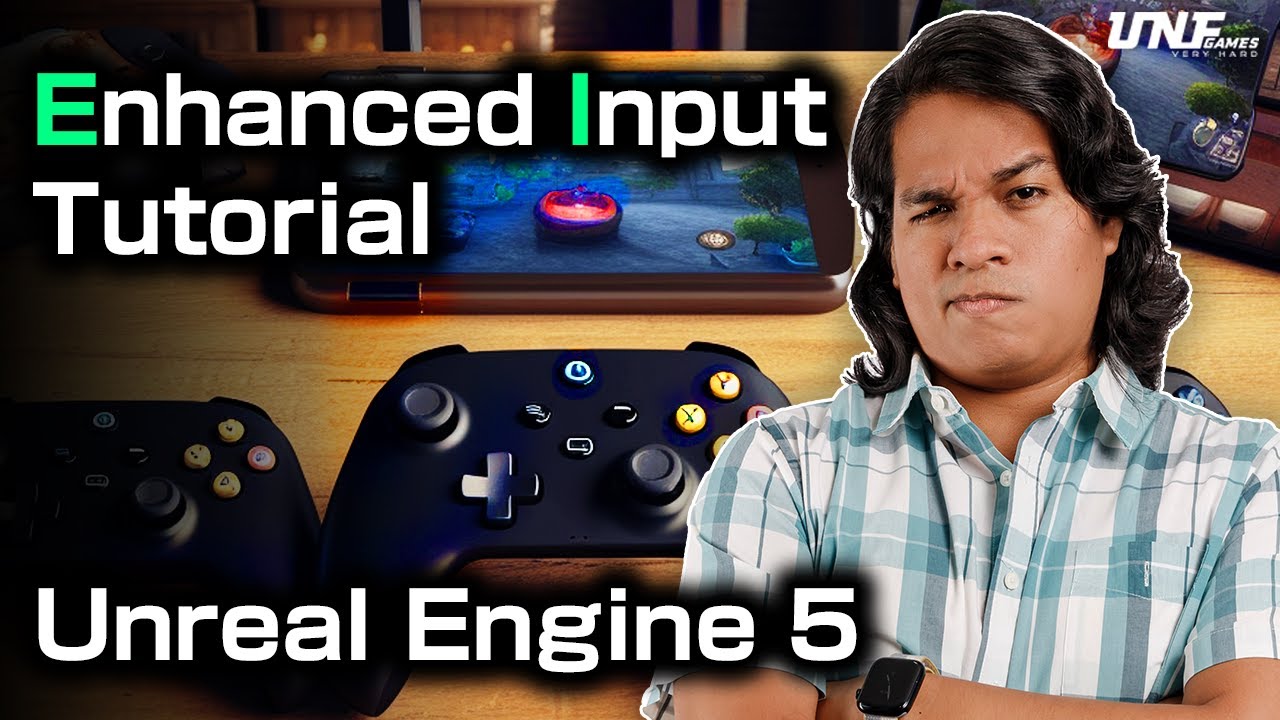
2:20:54
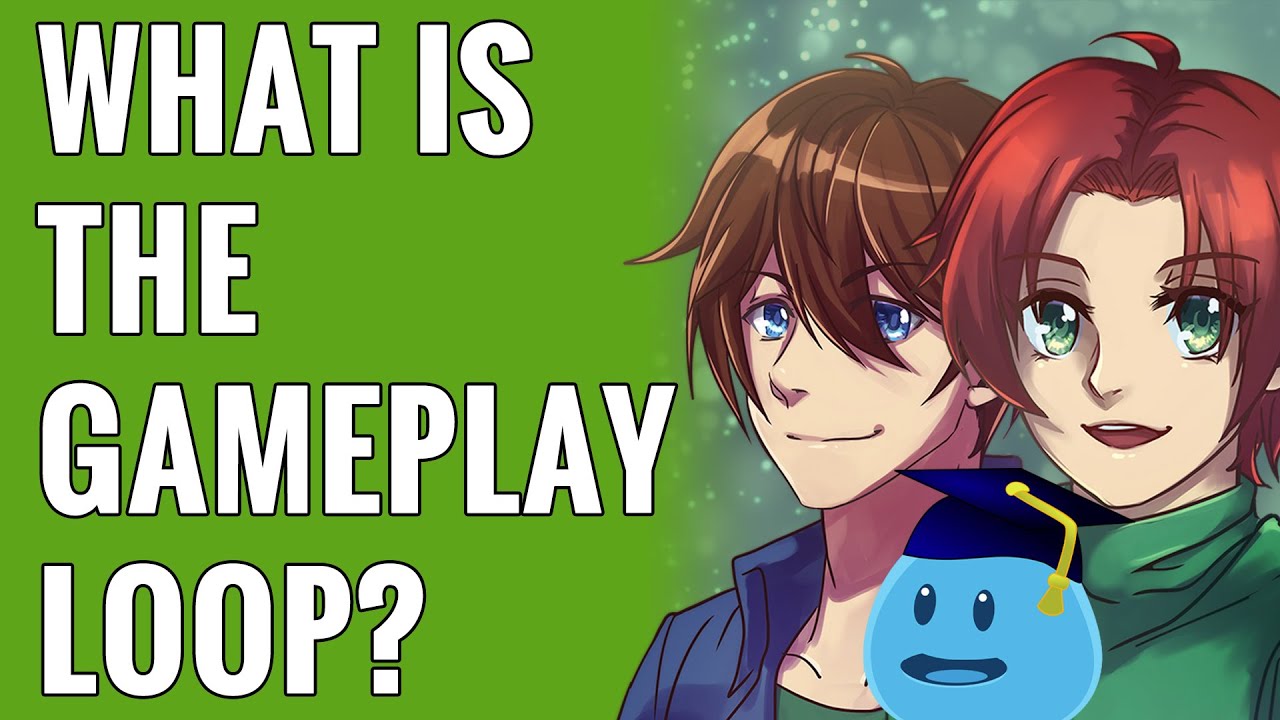
1:26:05
Character Movement
Player Control
The most basic aspect of character movement is how the player controls the character. This includes movement using keys or a controller thumbstick, as well as jumping, crouching, and sprinting.
Collision Detection
Physics algorithms detect when the character collides with objects, walls, or other characters. This ensures that characters cannot move through solid objects and provides a realistic feel to the game world.
Collision detection is integral to Unreal Engine and is handled automatically based on the collision settings of your objects.
Collision Settings:
Each object in Unreal Engine has collision settings that determine how it interacts with other objects. You can set these settings in the object’s Details panel, under the Collision category.
Collision Channels:
You can define custom collision channels with specific behaviors to control how objects interact with each other. This is useful for fine-tuning collision detection for specific interactions.
Character Collision:
For characters, the Character Movement Component has collision-related settings. These include settings for capsule collision, step height, and more.
Collision Responses:
You can define how objects respond to collisions, including whether they block, overlap, or ignore other objects with specific collision channels.
Acceleration and Deceleration
Characters often accelerate and decelerate gradually, mimicking real-world movement. This prevents abrupt starts and stops and adds to the sense of immersion.
Acceleration settings are primarily adjusted within the Character Movement Component.
Character Acceleration:
In the Character Movement Component, you can set various properties related to acceleration, such as Acceleration, Max Walk Speed, Air Control, and Ground Friction.
Object Acceleration:
For objects with physics, you can set their mass and physical properties, which will affect how they accelerate and respond to forces.
Jumping and Gravity
Jumping mechanics include jump height, hang time, and gravity’s effect on the character’s trajectory. These mechanics can be adjusted to suit the game’s style, from realistic to exaggerated.
Environmental Physics
Gravity
The strength of gravity in the game world affects how characters and objects fall and interact with the ground. Different dimensions or worlds within the game may have altered gravity, adding complexity to movement.
To set up gravity, you’ll typically work with the World Settings or Character Movement Component for your characters.
World Gravity (Project-Wide):
- In the main menu, go to Edit > Project Settings.
- In the left pane, expand Engine and select Physics.
- You can set the Gravity Z value to determine the strength and direction of gravity affecting all objects in the world.
Character-Specific Gravity:
- If you want to adjust gravity for specific characters, open your character’s Blueprint.
- In the Character Movement Component, find the “Falling” category.
- Set the Gravity Scale property to adjust the character’s gravity influence. A value of 2, for example, doubles the gravity force, while 0 disables gravity.
Friction
Friction determines how characters slide or stop when moving on surfaces. It’s crucial for realistic movement and can vary depending on the type of ground (e.g., ice, sand, concrete).
Friction settings can be adjusted within the Character Movement Component or the Physical Material assigned to objects.
Character Friction:
In the Character Movement Component, find the “Walking” category.
Adjust the properties like Braking Friction and Ground Friction to control how quickly the character comes to a stop or how much friction affects movement.
Object Friction:
You can assign Physical Materials to objects in your level.
Create or select a Physical Material Asset and adjust its friction values. Objects using this material will adhere to these friction settings.
Physics-Based Puzzles
Many games incorporate physics-based puzzles that require players to leverage environmental physics to solve challenges. These puzzles may involve manipulating objects, leveraging physics-based contraptions, or using gravity in creative ways.
Object Interaction
Pushing and Pulling
Characters may interact with objects in the game world by pushing or pulling them. The mechanics for how characters interact with these objects and their weight affect gameplay.
Object Physics
Objects in the game world often have their own physics properties, such as weight, mass, and rigidity. These properties determine how they react to external forces and collisions.
Object Interactions in Unreal Engine
1. Object Blueprint Setup:
Create a Blueprint for each interactable object in your game. To do this:
- Right-click in the Content Browser.
- Choose “Blueprint Class” and select “Actor” as the parent class.
- Name the Blueprint appropriately (e.g., “Interactable_Object_BP”).
Customize the Blueprint by adding a Static Mesh Component or any relevant components to represent the object’s visual and physical aspects.
Add a Collision Component (e.g., a Box Collision or Sphere Collision) to the Blueprint. This collision component will define the interaction area of the object.
2. Define Interaction Components:
- Inside the Blueprint, you can create custom components or use existing ones to define how the object can be interacted with. Common interaction components include:
- Interactive Component: A component that handles interactions with the object. This could be a sphere trigger, for example, which activates when the player approaches the object.
- Pickup Component: If the object is meant to be picked up, you can create a component that specifies the object’s pickup behavior.
3. Implement Object Interactions:
- Within the Blueprint’s Event Graph, set up functions or events that determine what happens when the object is interacted with.
- For example, if it’s a pickup object, create a function that triggers when the player interacts with it. This function might disable the object’s rendering, add it to the player’s inventory, and play a sound effect.
4. Input Setup:
- Define how the player will trigger object interactions. This typically involves setting up key bindings or input events that allow the player to interact with objects.
- You can use Unreal Engine’s Input system to capture player input for interaction actions.
5. Player Character Interaction:
- Implement interaction logic in the player character’s Blueprint or controller:
- When the player character overlaps with an interactable object’s collision volume, you can check for input (e.g., pressing a designated button) to trigger the interaction.
6. Feedback and Animation:
- Add feedback to indicate to the player that they can interact with an object. This could be a hover effect, a highlight, or a tooltip.
- You can also incorporate animations to make interactions feel more immersive. For example, a character reaching out to pick up an object.
7. Testing and Iteration:
- Test the interactions thoroughly to ensure they work as expected. Debug any issues that arise during testing and iterate on your Blueprint and interaction logic as needed.
8. Multiplayer Considerations (Optional):
- If your game is multiplayer, ensure that object interactions are properly replicated to all clients to maintain consistency across the network.
By following these steps, you can set up object interactions in Unreal Engine, allowing players to engage with objects in your game world. The specific details of your interactions will depend on your game’s design and requirements, so you may need to customize and extend these steps to suit your project’s needs.
Ragdoll Physics
Character Ragdoll
In some games, characters have ragdoll physics, meaning their bodies react realistically to forces and collisions. This can lead to dynamic and often comical character animations.
Death Animations
Ragdoll physics can be used to create lifelike death animations, making character deaths more impactful and realistic.
Environmental Interactions
Destructible Terrain
In games with destructible terrain, the environment responds to explosions, gunfire, or other destructive forces. This adds an extra layer of realism and interactivity to the world.
Dynamic Objects
ome objects in the game world can be moved, toppled, or altered by player actions. For example, pushing over a bookshelf to create a bridge or rolling a boulder to clear a path.
Climbing and Parkour
Climbing Mechanics
In some games, characters can climb ledges, walls, or structures. Climbing mechanics can be realistic or exaggerated, depending on the game’s style.
Parkour Elements
Games may incorporate parkour or free-running mechanics, allowing characters to perform acrobatic moves and navigate the environment dynamically.
Articles
Playlist
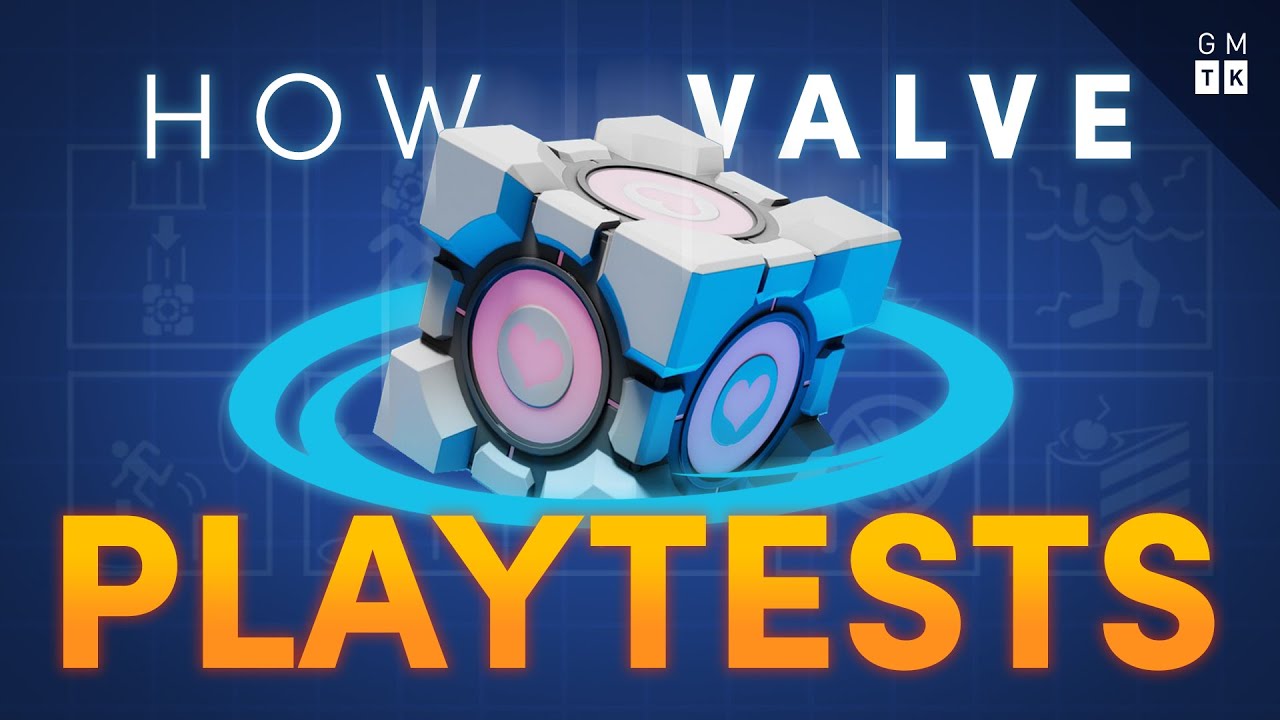
17:32
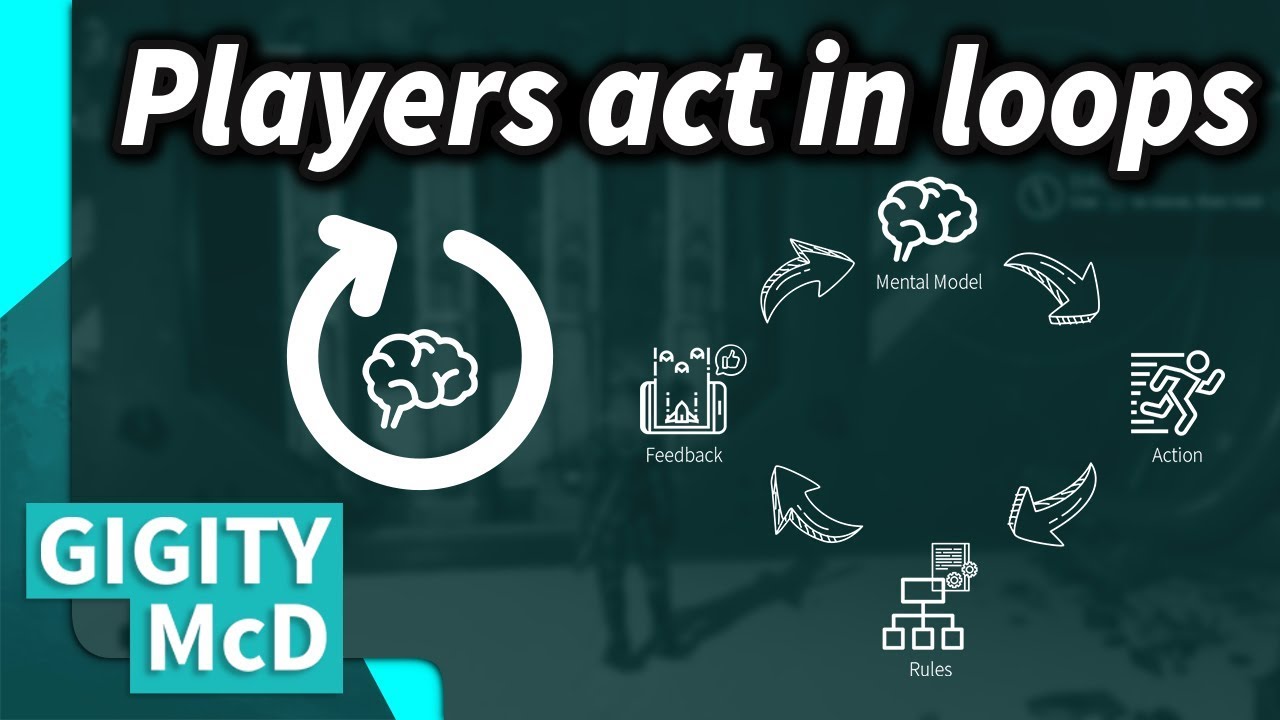
9:44
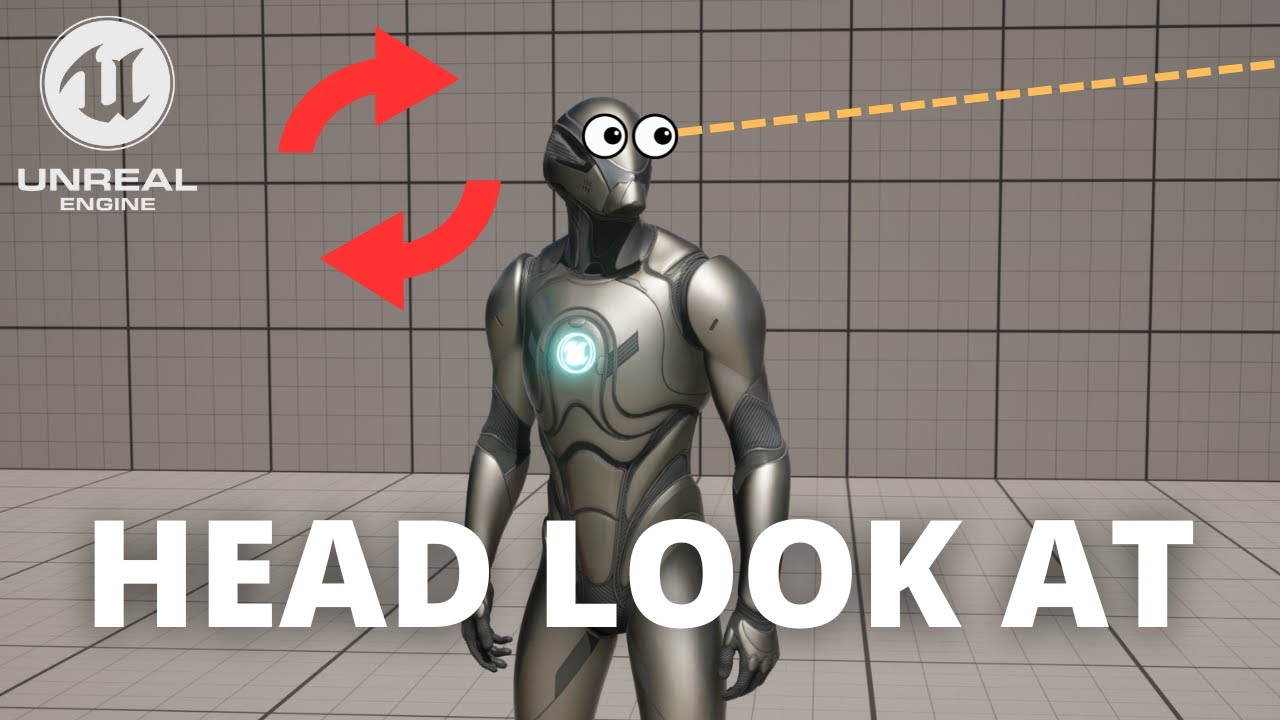
4:54
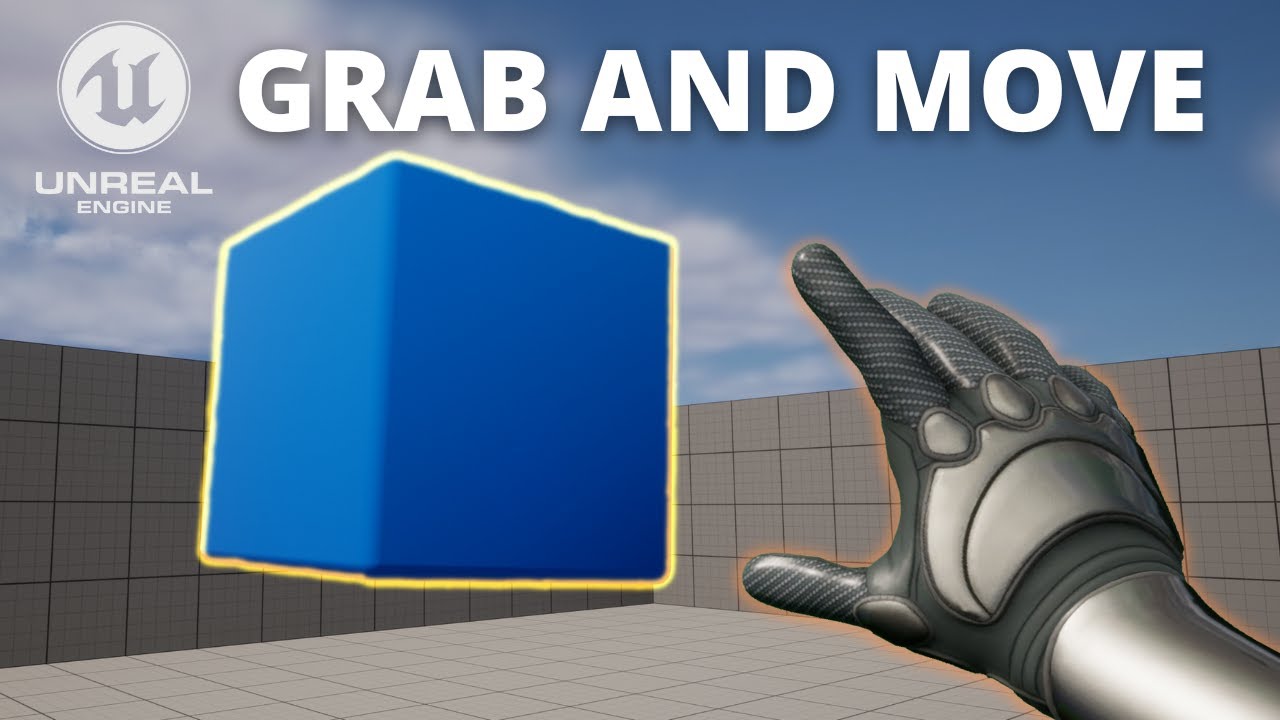
5:52
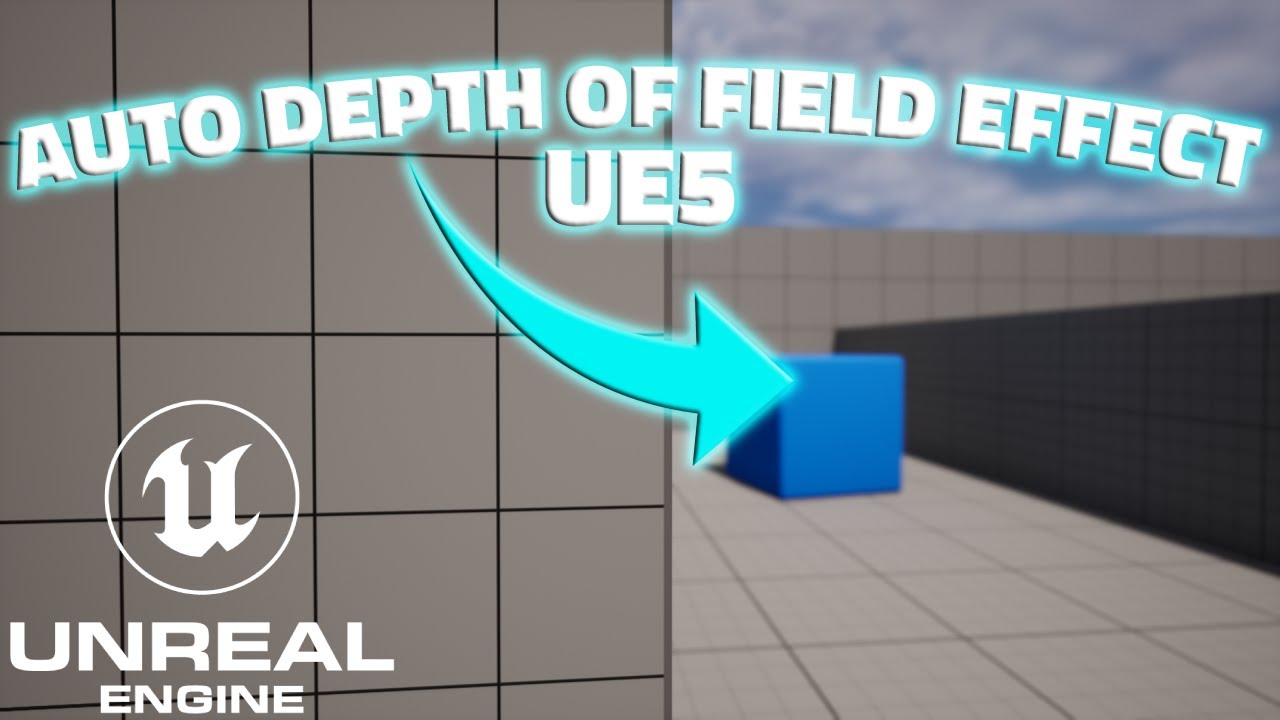
7:19
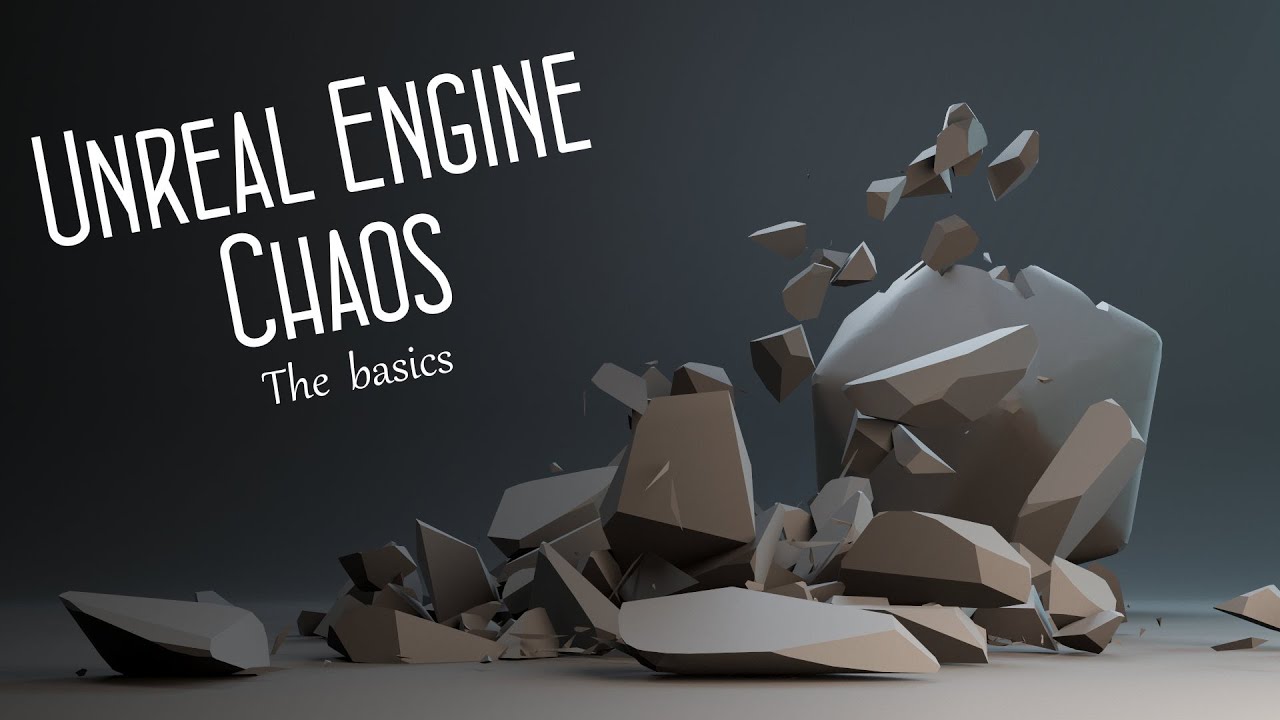
13:47
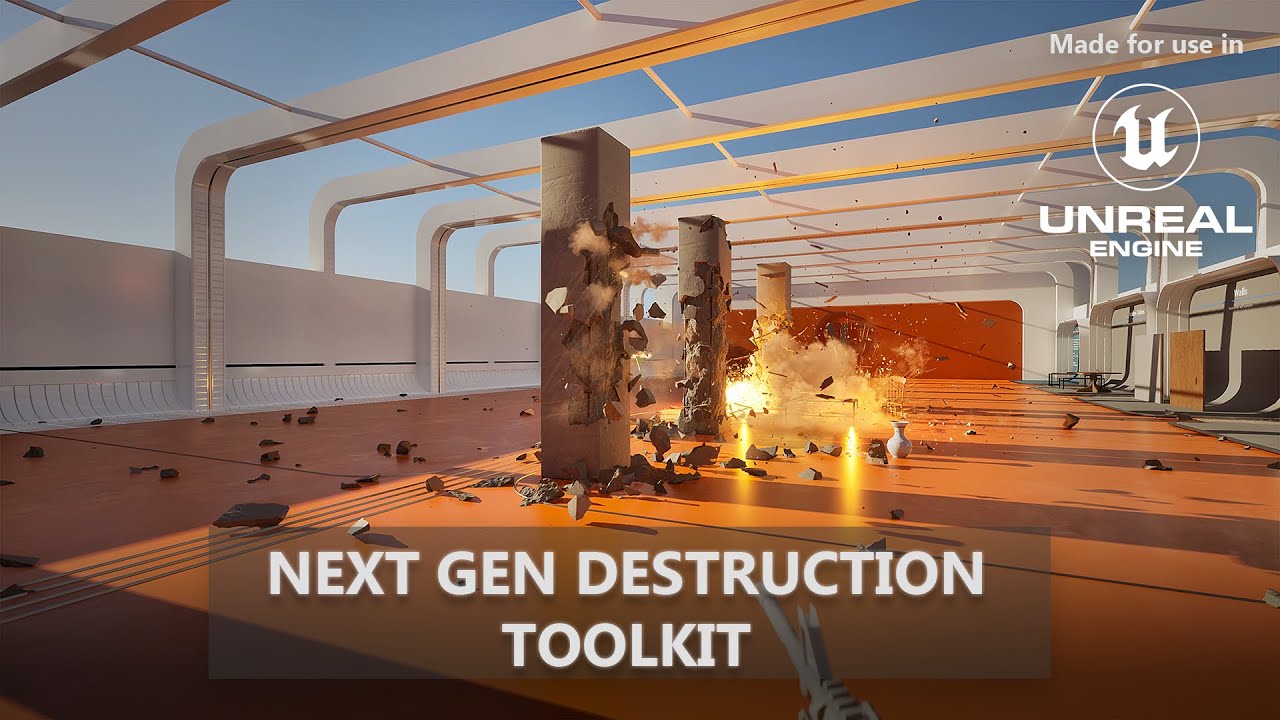